Go NLP API | Build Your Own
In my previous posts, I have talked about building a small backend server to handle HTTP POST request. We also have tried out the Google NLP and we dived into the topic of NLTK. Well, now we know how to create a Go
function to handle HTTP request and we understand the idea of Natural language Processing. Why not building your own Google NLP API?
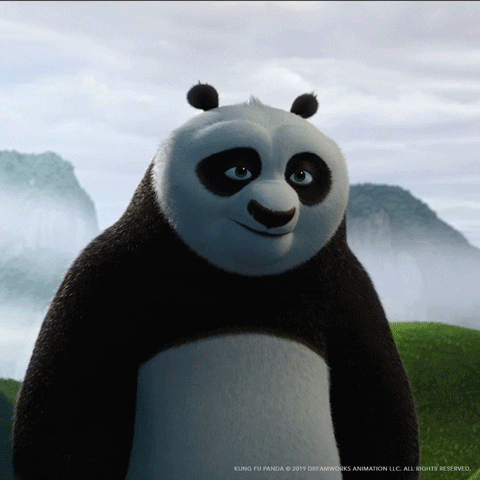
Let’s now look at the key functions
Let’s think about the API response data structure
Okie, dokie. Looks good! Now you will need a Golang backend to handle the http request
Oh! Yes! All set! Let’s start the http server!
Yes! Here you go! Your own nlp-api is up :)
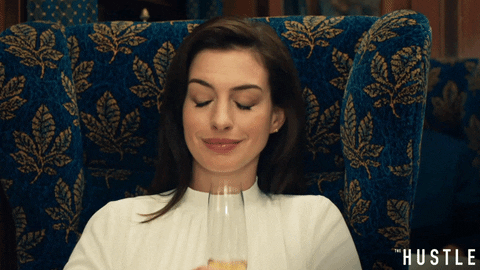
{“WhoamI”:“👋 from Pingzhou| ⛵”,“Tag”:[[“Google”,“NNP”],[",",","],[“headquartered”,“VBD”],[“in”,“IN”],[“Mountain”,“NNP”],[“View”,“NNP”],["(","("],[“1600”,“CD”],[“Amphitheatre”,“NNP”],[“Pkwy”,“NNP”],[",",","],[“Mountain”,“NNP”],[“View”,“NNP”],[",",","],[“CA”,“NNP”],[“940430”,“CD”],[")",")"],[",",","],[“unveiled”,“VBD”],[“the”,“DT”],[“new”,“JJ”],[“Android”,“NNP”],[“phone”,“NN”],[“for”,“IN”],[“$”,“$”],[“799”,“CD”],[“at”,“IN”],[“the”,“DT”],[“Consumer”,“NNP”],[“Electronic”,“NNP”],[“Show.",“NNP”],[“Sundar”,“NNP”],[“Pichai”,“NNP”],[“said”,“VBD”],[“in”,“IN”],[“his”,“PRP$”],[“keynote”,“NN”],[“that”,“IN”],[“users”,“NNS”],[“love”,“VBP”],[“their”,“PRP$”],[“new”,“JJ”],[“Android”,“NNP”],[“phones”,“NNS”],[".","."]],“Ent”:[[“Google”,“GPE”],[“Mountain View”,“GPE”],[“Mountain View”,“GPE”],[“Consumer Electronic Show. Sundar Pichai”,“ORGANIZATION”]]}
[ “Google”, “GPE” ] [ “Mountain View”, “GPE” ] [ “Mountain View”, “GPE” ] [ “Consumer Electronic Show. Sundar Pichai”, “ORGANIZATION” ]
[ “Google”, “NNP” ]